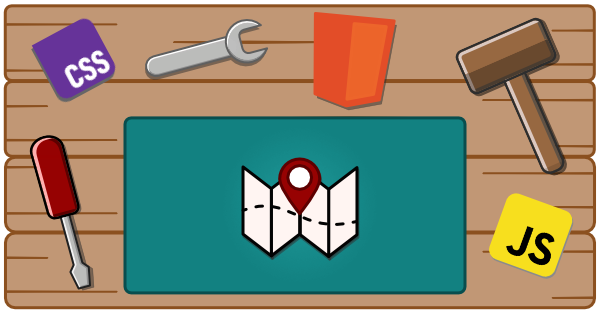
Module Path Resolver
Update module paths in your Custom Elements Manifest to the output target
This tool is designed to update module paths in your Custom Elements Manifest to the output target.
The goal is to make the paths in the CEM as accurate as possible so tools can accurately reference these.
Installation
To install the package, use the following command:
npm install -D @wc-toolkit/module-path-resolver
Usage
This package includes two ways to update the Custom Elements Manifest:
- using it in a script
- as a plugin for the Custom Element Manifest Analyzer.
Script
import { resolveModulePaths, type ModulePathResolverOptions } from "@wc-toolkit/module-path-resolver";import manifest from "./path/to/custom-elements.json" with { type: 'json' };
const options: ModulePathResolverOptions = {...};
resolveModulePaths(manifest, options);
CEM Analyzer
The plugin can be added to the Custom Elements Manifest Analyzer configuration file.
import { modulePathResolverPlugin } from "@wc-toolkit/module-path-resolver";
const options = {...};
export default { plugins: [ modulePathResolverPlugin(options) ],};
Configuration
type ModulePathResolverOptions = { /** The template for creating the component's import path */ modulePathTemplate?: ( /** The current value in the `path` property (typically to the source code) */ modulePath: string, /** The name of the component */ name: string, /** The tag name of the component */ tagName?: string ) => string; /** The template for creating the component's import path */ definitionPathTemplate?: ( /** The current value in the `path` property (typically to the source code) */ modulePath: string, /** The name of the component */ name: string, /** The tag name of the component */ tagName?: string ) => string; /** The template for creating the component's import path */ typeDefinitionPathTemplate?: ( /** The current value in the `path` property (typically to the source code) */ modulePath: string, /** The name of the component */ name: string, /** The tag name of the component */ tagName?: string ) => string; /** Path to output directory */ outdir?: string; /** The of the loader file */ fileName?: string; /** Class names of any components you would like to exclude from the custom data */ exclude?: string[]; /** Enables logging during the component loading process */ debug?: boolean; /** Prevents plugin from executing */ skip?: boolean;};
Module Path
This is the path to the component’s final output location.
{ // ex: module moved from `./src/components/alert/alert.ts` => `./dist/components/alert/alert.js` modulePathTemplate(modulePath, name, tagName) => modulePath.replace('src', 'dist').replace('.ts', '.js');
// ex: module moved from `packages/my-button/src/component/my-button.ts` => `dist/components/my-button.js` modulePathTemplate(modulePath, name, tagName) => `./dist/components/${tagName}.js`;
// ex: similar components are now grouped into the same module modulePathTemplate(modulePath, name, tagName) => { switch (tagName) { case 'my-tab': case 'my-tabs': case 'my-tabpanel': return `./dist/components/tabs/${className}.js`; case 'my-select': case 'my-option': return `./dist/components/select/${className}.js`; default: return `./dist/components/${tagName}/${className}.js`; } }}
Definition Path
This is the path to where the component is defined either through the traditional method (customElements.define('my-component', MyComponent)
), a decorator, or some other custom approach.
{ definitionPathTemplate(modulePath, name, tagName) => `./dist/${tagName}/index.js`}
Type Definition Path
This sets a non-standard property next to the module path so tools can easily reference them.
{ typeDefinitionPathTemplate(modulePath, name, tagName) => `./types/components/${tagName}.d.ts`}