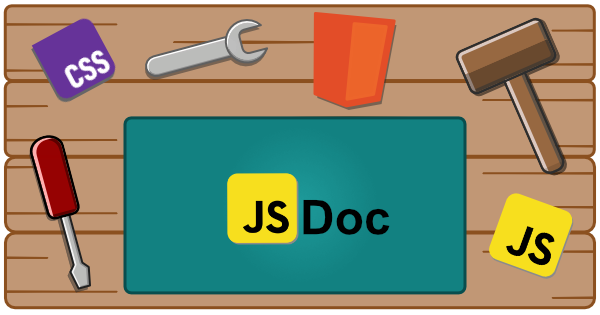
Custom JSDoc Tags
This is a plugin maps custom JSDoc tags on your component classes to properties in Custom Elements Manifest using the Custom Element Manifest Analyzer.
Installation
npm i -D @wc-toolkit/jsdoc-tags
Usage
Add the information you would like to include with you component in the class’s JSDoc comment using custom tags. In this example, the @dependency
, @since
, @status
, and @spec
tags are all custom.
/** * * My custom element does some amazing things * * @tag my-element * * @dependency icon * @dependency button * * @since 1.2.5 * * @status beta - not ready for production * * @spec https://www.figma.com/... * */export class MyElement extends HTMLElement { ...}
In the CEM analyzer config, import the plugin and add the mappings for the new tags.
import { jsDocTagsPlugin } from "@wc-toolkit/jsdoc-tags";
export default { ... /** Provide custom plugins */ plugins: [ jsDocTagsPlugin({ tags: { // finds the values for the `@since` tag since: {}, // finds the values for the `@status` tag status: {}, // finds the values for the `@spec` tag spec: {}, // finds the values for the `@dependency` tag dependency: { // maps the values to the `dependencies` property in the CEM mappedName: 'dependencies', // ensures the values are always in an array (even if there is only 1) isArray: true, }, } }), ],};
Result
The data should now be included in the Custom Elements Manifest.
{ "kind": "class", "description": "My custom element does some amazing things", "name": "MyElement", "tagName": "my-element", "since": { "name": "1.2.5", "description": "" }, "status": { "name": "beta", "description": "not ready for production" }, "spec": { "name": "https://www.figma.com/...", "description": "" }, "dependencies": [ { "name": "icon", "description": "" }, { "name": "button", "description": "" } ]}
How It Works
When the analyzer encounters a specified tag, it will add it to a corresponding property in the Custom Elements Manifest for the class with the following data (depending on what you have specified in your tag).
/** * * @custom tag1 - This tag does something incredible * */export class MyElement extends HTMLElement {}
{ "custom": { "name": "tag1", "description": "This tag does something incredible" }}
For you TypeScript fans out there…
/** Configuration for the individual tag */type CustomTag = { /** The name of the tag to be parsed */ [key: string]: { /** The name of the property to be added to the CEM */ mappedName?: string; /** The type of the property to be added to the CEM */ isArray?: boolean; };};
If it encounters another tag by the same name, it will convert the value to an array and add the new tag information to it.
/** * * @custom tag1 - This tag does something incredible * @custom tag2 - This tag also does something incredible * */export class MyElement extends HTMLElement {
{ "custom": [ { "name": "tag1", "description": "This tag does something incredible" }, { "name": "tag2", "description": "This tag also does something incredible" } ]}
The tag accepts the standard JSDoc syntax so you can specify a name, type, default value, and description.
/** * @custom {string | undefined} [tag1=Hello world!] - This tag does something incredible */
{ "custom": { "name": "tag1", "type": { "text": "string | undefined" }, "default": "Hello world!", "description": "This tag does something incredible" }}
Configuration
The plugin takes the following options:
/** Configuration for the CEM plugin */type Options = { /** The name of the tags to be parsed and their configuration */ tags?: CustomTag; /** Show process logs */ debug?: boolean; /** Prevents plugin from executing */ skip?: boolean;};
Tags
The property used to identify the configuration for your JSDoc is the name of the tag you are using in your component. If you don’t have any configurations for the tag, you can just add an empty object as the property value.
/** * * @since 1.2.5 * */export class MyElement extends HTMLElement {}
export default { ... plugins: [ customJSDocTagsPlugin({ tags: { since: {}, } }), ],};
{ "name": "MyElement", "since": { "name": "1.2.5", "description": "" }}
Mapped Name
If you need the property name in the custom Elements Manifest to differ from the JSDoc name, you can use the mappedName
setting. This is helpful for tags that are singular, but will be accumulated into an array in the manifest, so you want to make the property name plural.
/** * * @dependency icon * @dependency button * */export class MyElement extends HTMLElement {}
export default { ... plugins: [ customJSDocTagsPlugin({ tags: { dependency: { mappedName: 'dependencies', }, } }), ],};
{ "name": "MyElement", "dependencies": [ { "name": "icon", "description": "" }, { "name": "button", "description": "" } ]}
Is Array
If you would always like the property value in the manifest to always be an array - even if there is a single value, setting the isArray
value to true
will convert the value to an array.
export default { ... plugins: [ customJSDocTagsPlugin({ tags: { dependency: { mappedName: 'dependencies', isArray: true, }, } }), ],};
/** * * @dependency icon * */export class MyElement extends HTMLElement {
{ "name": "MyElement", "dependencies": [ { "name": "icon", "description": "" } ]}